CMSC 132A Lecture Notes - Lecture 17: String Literal, Character Class
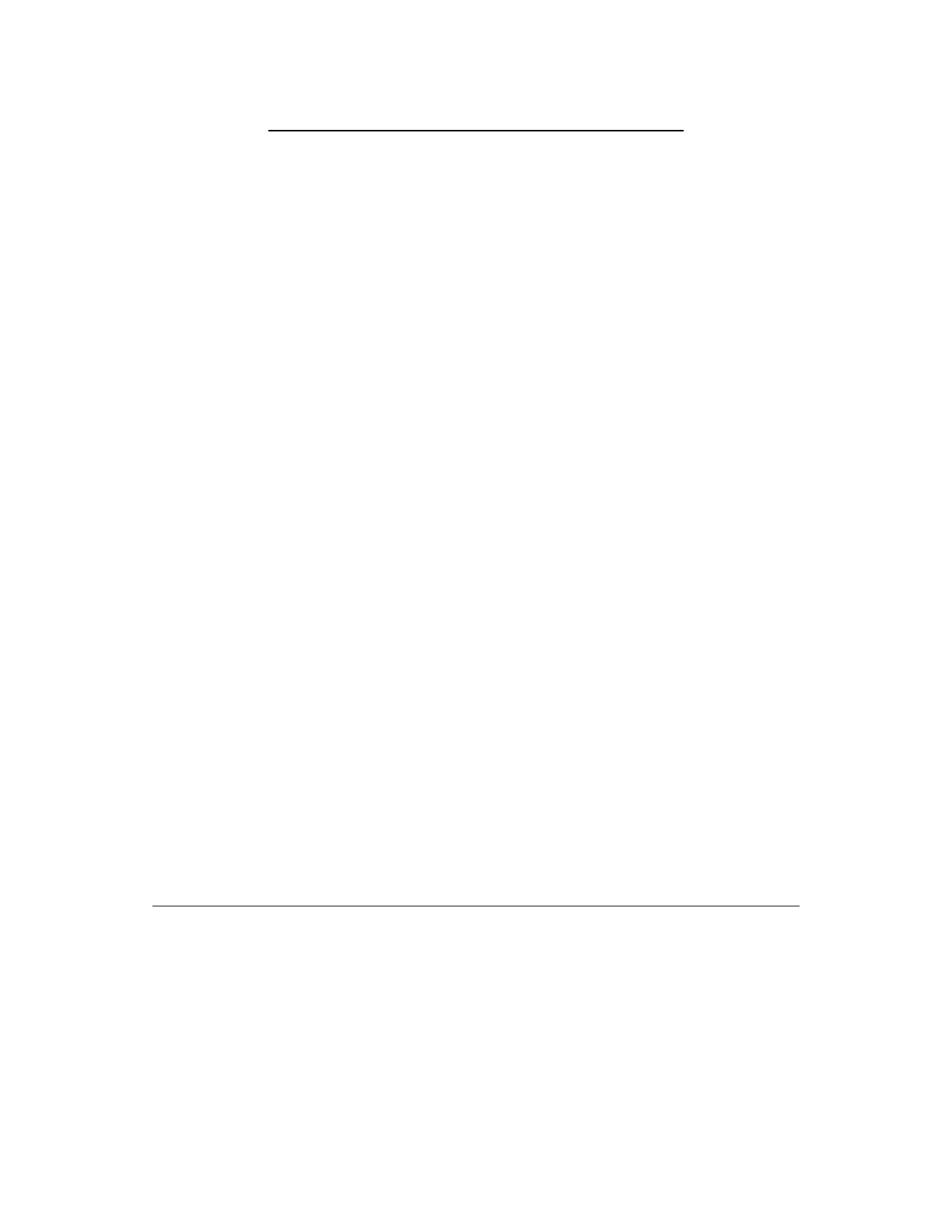
CMSC132A Lecture 17: Characters and Strings
Most of the time, if you are using a single character value, you will use the primitive char
type. For example:
char ch = 'a';
// Unicode for uppercase Greek omega character
char uniChar = '\u03A9';
// an array of chars
char[] charArray = { 'a', 'b', 'c', 'd', 'e' };
There are times, however, when you need to use a char as an object—for example, as
a method argument where an object is expected. The Java programming language
provides a wrapper
class that "wraps" the charin a Character object for this purpose. An
object of type Character contains a single field, whose type is char. This Character class
also offers a number of useful class (i.e., static) methods for manipulating characters.
You can create a Character object with the Character constructor:
Character ch = new Character('a');
The Java compiler will also create a Character object for you under some
circumstances. For example, if you pass a primitive char into a method that expects an
object, the compiler automatically converts the charto a Character for you. This feature
is called autoboxing
—or unboxing
, if the conversion goes the other way. For more
information on autoboxing and unboxing, see Autoboxing and Unboxing.
Note: The Character class is immutable, so that once it is created, a Character
object cannot be changed.
Strings
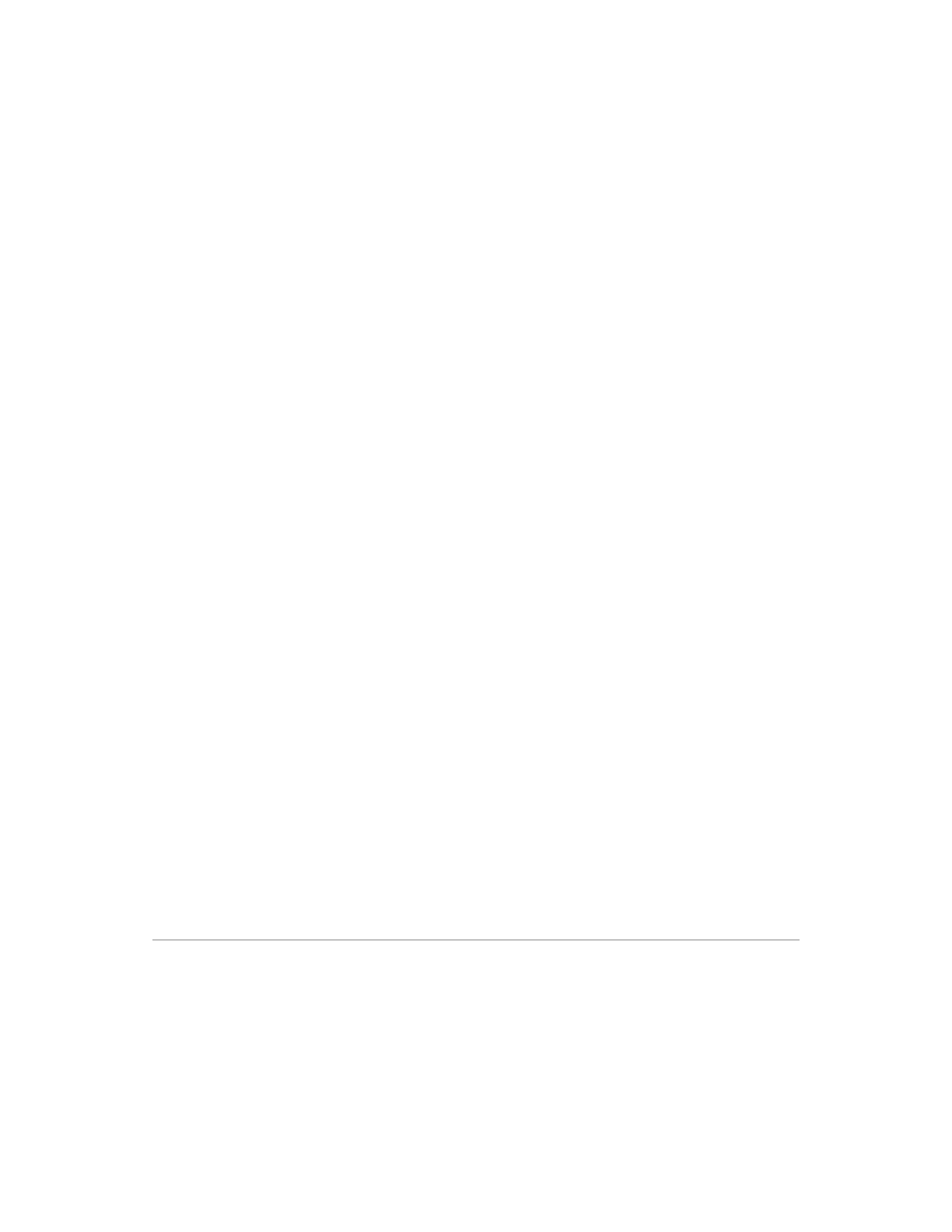
Strings, which are widely used in Java programming, are a sequence of characters.
In the Java programming language, strings are objects.
The Java platform provides the String class to create and manipulate strings.
Creating Strings
The most direct way to create a string is to write:
String greeting = "Hello world!";
In this case, "Hello world!" is a string literal
—a series of characters in your code that
is enclosed in double quotes. Whenever it encounters a string literal in your code,
the compiler creates a String object with its value—in this case, Hello world!.
As with any other object, you can create String objects by using the new keyword
and a constructor. The String class has thirteen constructors that allow you to
provide the initial value of the string using different sources, such as an array of
characters:
char[] helloArray = { 'h', 'e', 'l', 'l', 'o', '.' };
String helloString = new String(helloArray);
System.out.println(helloString);
The last line of this code snippet displays hello.
Note: The String class is immutable, so that once it is created a String object
cannot be changed. The String class has a number of methods, some of which
will be discussed below, that appear to modify strings. Since strings are
Document Summary
Most of the time, if you are using a single character value, you will use the primitive char type. // unicode for uppercase greek omega character char unichar = "\u03a9"; // an array of chars char[] chararray = { "a", "b", "c", "d", "e" }; There are times, however, when you need to use a char as an object for example, as a method argument where an object is expected. The java programming language provides a wrapper class that wraps the charin a character object for this purpose. An object of type character contains a single field, whose type is char. This character class also offers a number of useful class (i. e. , static) methods for manipulating characters. You can create a character object with the character constructor: The java compiler will also create a character object for you under some circumstances.