CSE 14 Lecture 6: CMPS 12A Lecture 6: Iterative Control: For, While, and Do-While Loops
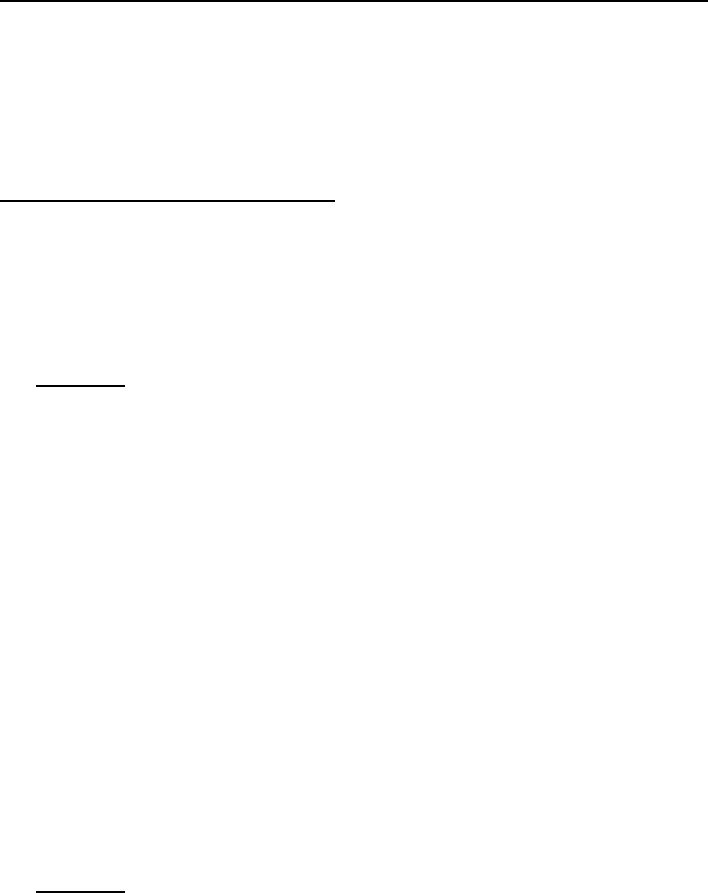
CMPS12A Lecture 6 – Iterative Control: For, While, and Do-While Loops
In good programming, loops are essential in getting what you need. Combined with the
conditional operations, you can easily take control of your program and make it go a certain
direction depending on those conditions. I am going to tell you that the while and for loop are
the most used loops because they are easy to do and more understandable.
Iterative control structures (loops)
- while
- for
- do-while
Example:
while (cond) { //(cond) would be the loop repetition cond (LRC)
stmnt;
stmnt; //inside here would be the loop body
…
stmnt;
}
stmnt;
…
Again, if the loop body only consists of one line of code, the braces {} are optional.
When using a while loop for iterations and looping, you will need to make a loop control
variable, first and foremost, outside the while loop. Look at this example:
Example:
int i = 0; //loop control variable (LCV)
while ( i < 10) { //this is the LRC
System.out.println(i);
i++; //increment LCV
}
I made an integer variable i initiated to 0. I then implemented a while loop that prints out i
and increments i every time it is at the end of the loop until i is finally less than 10. The
output would thus be the numbers from 0 through 9 in each line. If we initiated int i inside
the while loop and made the hile oditio i < , it ould’t hae orked. Also otie
find more resources at oneclass.com
find more resources at oneclass.com

that I add the i++ at the end of the while loop for incrementing i at the end, beginning the
loop again with the next integer incremented.
I want to note that the example above is basically the same thing as this for-loop:
Example:
for (int i = 0; i < 10; i++) //basically this is the LCV followed by the LRC, and then inc. LCV
System.out.println(i);
The only difference here in the for-loop is that we write everything in the conditional loop in
for(). We initiate int i = 0 as the loop control variable, then use ; followed by i < 10, then use
; and finally the control variable i++ where it is incremented at the end of the loop. It is up
to the programmer in what they want to use, although there will be times where one loop is
better than the other.
The other loop that one can do is the do-while loop, which is almost the same thing as the
while loop. It looks something like this:
Example:
do {
stmnt;
stmnt;
…
stmnt;
}
while (cond); //this can also be next to the } above as this→ } while (cond);
stmnt;
…
Unlike the while loop, the do-while body must execute 1 time to be able to function
properly. Since do comes out first in the program, it has to do execute this, THEN it reads
the while condition and would repeat it again until that condition is met. Lets look at more
for-loop and while-loop examples:
Example:
import java.util.Scanner;
class Example1 {
public static void main(String [] args) {
find more resources at oneclass.com
find more resources at oneclass.com
Document Summary
Cmps12a lecture 6 iterative control: for, while, and do-while loops. In good programming, loops are essential in getting what you need. Combined with the conditional operations, you can easily take control of your program and make it go a certain direction depending on those conditions. I am going to tell you that the while and for loop are the most used loops because they are easy to do and more understandable. //(cond) would be the loop repetition cond (lrc) stmnt; stmnt; //inside here would be the loop body stmnt; stmnt; Again, if the loop body only consists of one line of code, the braces {} are optional. When using a while loop for iterations and looping, you will need to make a loop control variable, first and foremost, outside the while loop. Example: int i = 0; while ( i < 10) { I made an integer variable i initiated to 0.