CMPT 166 Lecture Notes - Lecture 15: Ellipse, The Nice, Thx
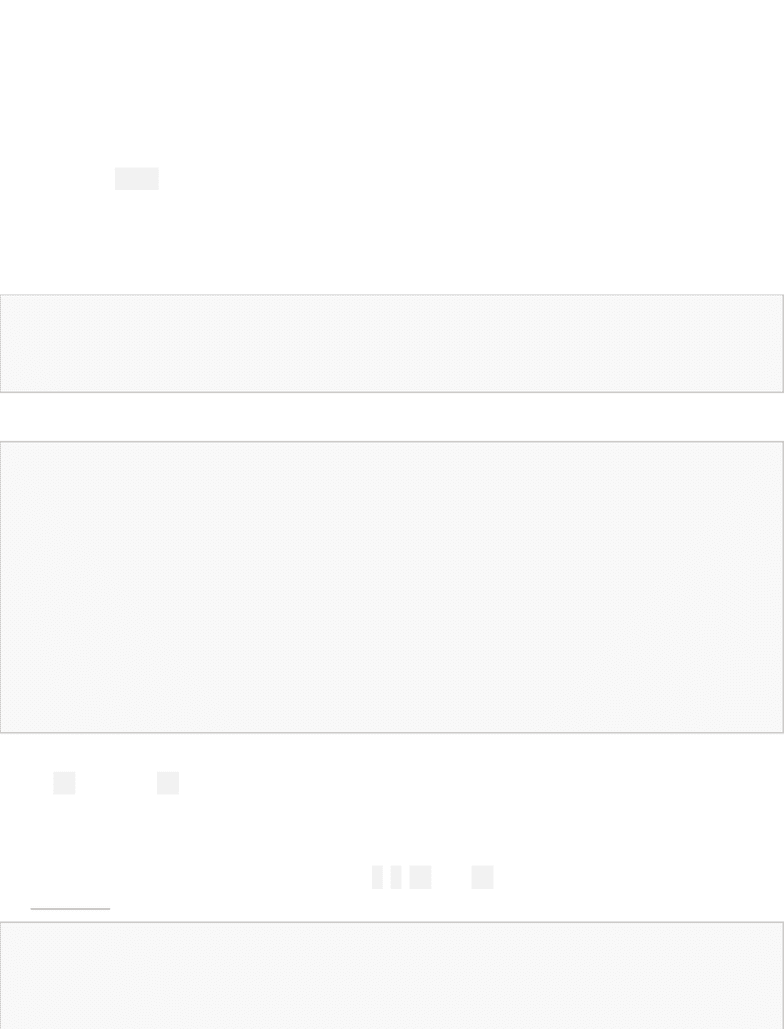
Sprites
In these notes you will learn:
• How to write a simple class.
• How to use objects with ”.”-notation.
• How assignment works with object variables.
• What null is.
Introduction
Every 2-dimensional animated object needs, at least, these four variables:
float x; // (x, y) is the position
float y; // of the object
float dx; // speed along the x-axis
float dy; // speed along the y-axis
So, for example, if you want to animated 3 different objects, you will need at least 12 variables:
float x1; // object 1
float y1;
float dx1;
float dy1;
float x2; // object 2
float y2;
float dx2;
float dy2;
float x3; // object 3
float y3;
float dx3;
float dy3;
Dealing with so many similarly-named variables is quite difficult in practice. It’s easy to accidentally
write y2 instead of y1.
Sprites
One way to deal with this complexity is to group x, y, dx, and dy together into one object. To do this
in Processing, we first need to create a class:
class Sprite {
float x;
float y;
float dx;
float dy;
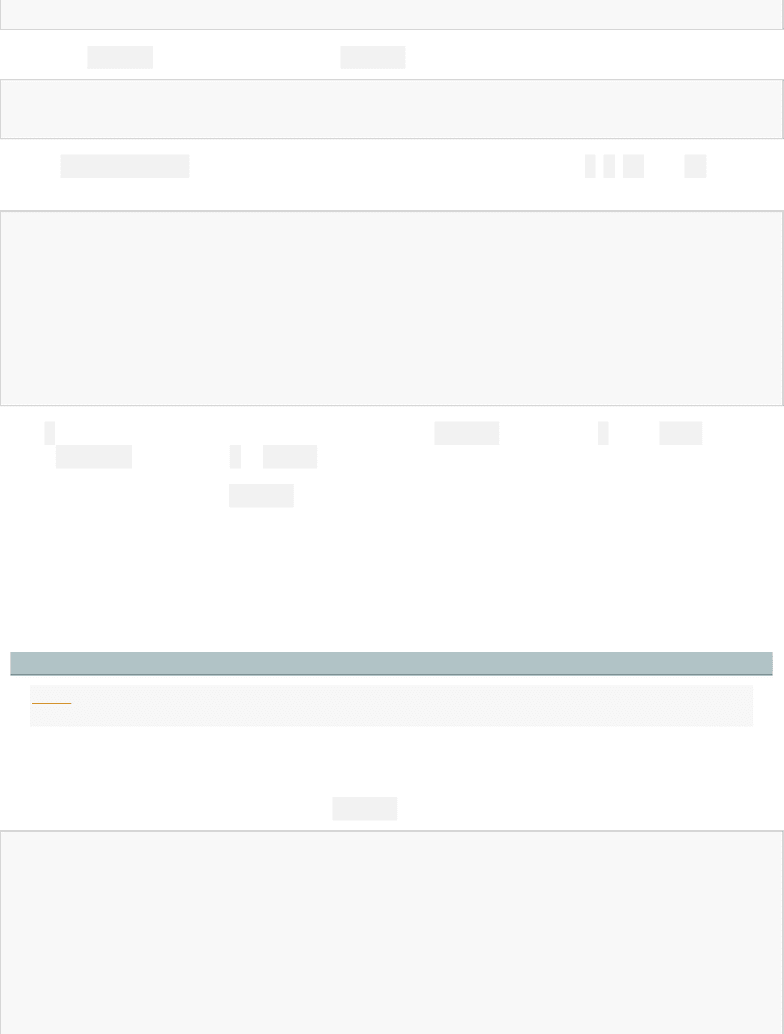
}
Using the Sprite class, we can now create Sprite objects like this:
Sprite ball = new Sprite();
Sprite plate = new Sprite();
When new Sprite() is called, it creates a new object that contains its own x, y, dx, and dy values.
We can use it like this:
ball.x = 10; // initialize ball
ball.y = 10;
ball.dx = 1;
ball.dy = 1.5;
plate.x = 450; // initialize plate
plate.y = 450;
plate.dx = -1.5;
plate.dy = -1;
The . is used to access the variables in an object. Clearly, ball.x refers to the x in the ball object,
while plate.x refers to the x in plate.
Two advantages of using the Sprite class are:
• The variable names tend to be more descriptive.
• You don’t have to define as many variables.
While this doesn’t solve all the problems of so many variables, it is an important first step. Later, we will
see how other features can be used to even further simplify the creation of animated objects.
Note
Sprite is the traditional name for a 2-dimensional animated object. It originated in early video games, where
support for animated objects was often designed into the hardware itself.
Sample Program
Here’s a complete sample program using a Sprite to represent a ball:
class Sprite {
float x;
float y;
float dx;
float dy;
}
color white = color(255);
color orange = color(255, 165, 0);
Document Summary
In these notes you will learn: how to write a simple class, how to use objects with . -notation, how assignment works with object variables, what null is. Every 2-dimensional animated object needs, at least, these four variables: float x; // (x, y) is the position float y; // of the object float dx; // speed along the x-axis float dy; // speed along the y-axis. Dealing with so many similarly-named variables is quite difficult in practice. It"s easy to accidentally write y2 instead of y1. One way to deal with this complexity is to group x, y, dx, and dy together into one object. To do this in processing, we first need to create a class: class sprite { float x; float y; float dx; float dy; Using the sprite class, we can now create sprite objects like this: When new sprite() is called, it creates a new object that contains its own x, y, dx, and dy values.